The Vertex Collection#
Warning
Not all examples on this page have been updated to r22 and ServiceX 3. Examples that have not been updated will be marked using a similar warning. These examples will be updated soon.
The PrimaryVertex
collection contains a list of all the primary vertices in the event. The first one is the Tracking group’s pick for the primary vertex.
from config import get_data
from config import sx_f
from func_adl_servicex_xaodr22 import FuncADLQueryPHYSLITE
import matplotlib.pyplot as plt
import numpy as np
import awkward as ak
Here we fetch all the primary vertices’s in an event, their location and how many tracks are attached to them. Just as in the previous chapter, we need to specify the bank name:
query = FuncADLQueryPHYSLITE()
vertices_per_event = (query
.Select(lambda e: e.Vertices("PrimaryVertices"))
.Select(lambda vert: {
'x': vert.Select(lambda v: v.x()),
'y': vert.Select(lambda v: v.y()),
'z': vert.Select(lambda v: v.z()),
'n': vert.Select(lambda v: v.nTrackParticles()),
})
)
vertices_data = get_data(vertices_per_event,sx_f)
plt.hist(ak.flatten(vertices_data.n), bins=50, range=(0, 100))
plt.xlabel('Number of tracks in each vertex')
plt.ylabel('Number of Vertices')
_ = plt.title('Number of tracks in each vertex for $Z\\rightarrow ee$ events')
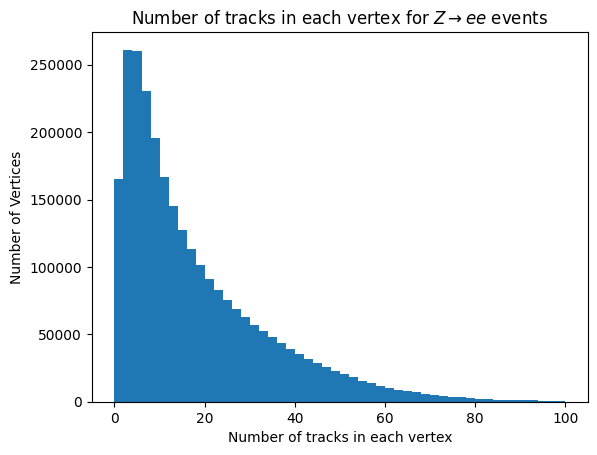
plt.scatter(ak.flatten(vertices_data.x), ak.flatten(vertices_data.y))
plt.xlabel('$v_x$ [mm]')
plt.ylabel("$v_y$ [mm]")
_ = plt.title('Vertex position for $Z\\rightarrow ee$ events')
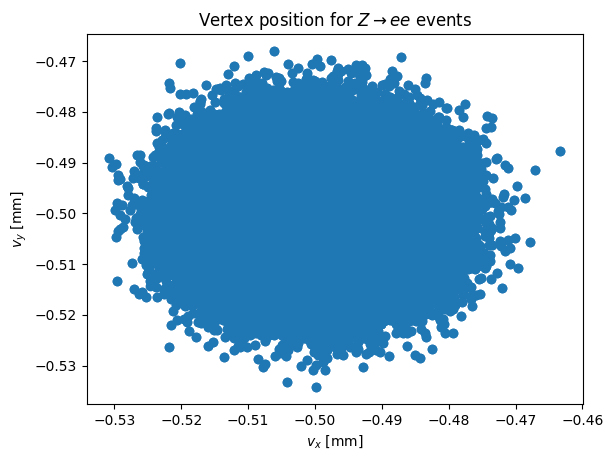
At the Tevatron you could see the beam tilt. No so much here at the LHC!
plt.scatter(ak.flatten(vertices_data.z),ak.flatten(vertices_data.y))
plt.xlabel('$v_z$ [mm]')
plt.ylabel("$v_y$ [mm]")
_ = plt.title('Vertex position for $Z\\rightarrow ee$ events')
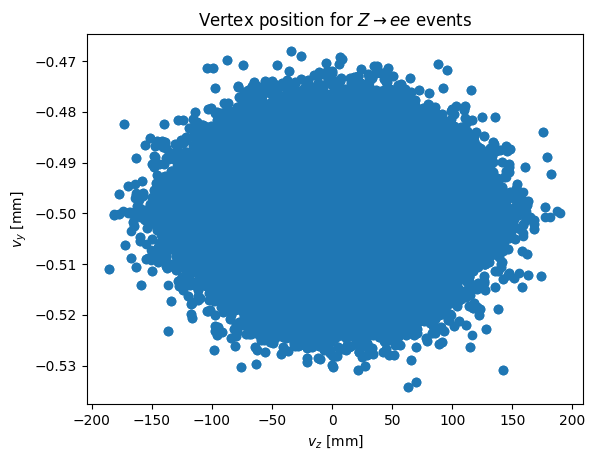
Normally one wants only the primary vertex - which in ATLAS is the first vertex in the collection.
Warning
This example has not been updated to r22 and ServiceX 3 yet.
pvs = (ds
.Select(lambda e: e.Vertices("PrimaryVertices").First())
.Select(lambda v:
{
"x": v.x(),
"y": v.y(),
"z": v.z(),
"n": v.nTrackParticles(),
})
.AsAwkwardArray()
.value())
---------------------------------------------------------------------------
NameError Traceback (most recent call last)
Cell In[6], line 1
----> 1 pvs = (ds
2 .Select(lambda e: e.Vertices("PrimaryVertices").First())
3 .Select(lambda v:
4 {
5 "x": v.x(),
6 "y": v.y(),
7 "z": v.z(),
8 "n": v.nTrackParticles(),
9 })
10 .AsAwkwardArray()
11 .value())
NameError: name 'ds' is not defined
plt.hist(pvs.n, bins=50, range=(0, 100))
plt.xlabel('Number of tracks in each primary vertex')
plt.ylabel('Number of Vertices')
_ = plt.title('Number of tracks in each PV for $Z\\rightarrow ee$ events')
---------------------------------------------------------------------------
ReturnValueException Traceback (most recent call last)
Cell In[15], line 1
----> 1 plt.hist(flat_array_from_files(files,'n'), bins=50, range=(0, 100))
2 plt.xlabel('Number of tracks in each primary vertex')
3 plt.ylabel('Number of Vertices')
File ~/WorkingDirectory/IrisHep/xaod_usage/book/chapters/config.py:90, in flat_array_from_files(files, branch_name)
88 def flat_array_from_files(files, branch_name):
89 data = []
---> 90 for file_path in files:
91 file = uproot.open(file_path)
92 array = file['atlas_xaod_tree;1'][branch_name].arrays(library="ak")[branch_name]
File /usr/lib64/python3.9/_collections_abc.py:984, in Sequence.__iter__(self)
982 try:
983 while True:
--> 984 v = self[i]
985 yield v
986 i += 1
File ~/.local/lib/python3.9/site-packages/servicex/servicex_client.py:79, in GuardList.__getitem__(self, index)
77 if not self.valid():
78 data = cast(Exception, self._data)
---> 79 raise data
80 else:
81 data = cast(Sequence, self._data)
ReturnValueException: Exception occurred while making ServiceX request.
Traceback (most recent call last):
File "/home/rjanusia/.local/lib/python3.9/site-packages/servicex/query_core.py", line 548, in as_files_async
return await self.submit_and_download(
File "/home/rjanusia/.local/lib/python3.9/site-packages/servicex/query_core.py", line 283, in submit_and_download
self.request_id = await self.servicex.submit_transform(sx_request)
File "/home/rjanusia/.local/lib/python3.9/site-packages/servicex/servicex_adapter.py", line 127, in submit_transform
raise RuntimeError("ServiceX WebAPI Error during transformation "
RuntimeError: ServiceX WebAPI Error during transformation submission: 500 - Something went wrong (Failed to generate translation code: Unable to generate a sequence from the given AST. Either there is an internal error, or you are trying to manipulate a xAOD::Vertex_v1* value (expression i_obj114) ('cpp_value') as a sequence (ast is: Call(func=Name(id='First', ctx=Load()), args=[Call(func=CPPCodeValue(), args=[Constant(value='PrimaryVertices')], keywords=[])], keywords=[])))
plt.scatter(pvs.x, pvs.y)
plt.xlabel('$v_x$ [mm]')
plt.ylabel("$v_y$ [mm]")
_ = plt.title('Primary Vertex position for $Z\\rightarrow ee$ events')
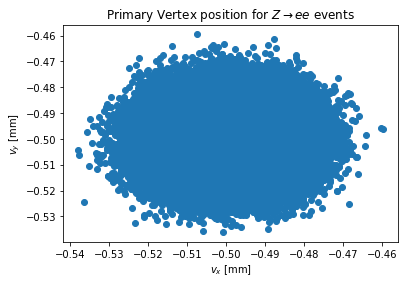
Note that the wings are gone now in the tilt plot, and the hight is more compressed. The wings were likely beam-gas interactions or similar.
plt.scatter(pvs.z, pvs.y)
plt.xlabel('$v_z$ [mm]')
plt.ylabel("$v_y$ [mm]")
_ = plt.title('Vertex position for $Z\\rightarrow ee$ events')
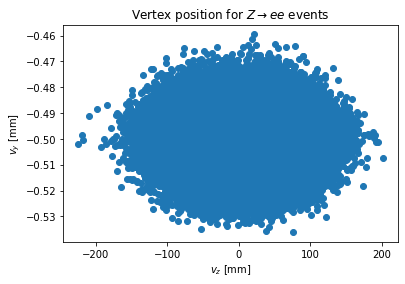
The Datamodel#
The data model when this documentation was last built was:
from func_adl_servicex_xaodr22.xAOD.trackparticle_v1 import TrackParticle_v1
help(TrackParticle_v1)
Help on class TrackParticle_v1 in module func_adl_servicex_xaodr22.xAOD.trackparticle_v1:
class TrackParticle_v1(builtins.object)
| A class
|
| Methods defined here:
|
| beamlineTiltX(self) -> 'float'
| A method
|
| beamlineTiltY(self) -> 'float'
| A method
|
| charge(self) -> 'float'
| A method
|
| chiSquared(self) -> 'float'
| A method
|
| clearDecorations(self) -> 'bool'
| A method
|
| d0(self) -> 'float'
| A method
|
| definingParametersCovMatrixDiagVec(self) -> 'func_adl_servicex_xaodr22.vector_float_.vector_float_'
| A method
|
| definingParametersCovMatrixOffDiagCompr(self) -> 'bool'
| A method
|
| definingParametersCovMatrixOffDiagVec(self) -> 'func_adl_servicex_xaodr22.vector_float_.vector_float_'
| A method
|
| definingParametersCovMatrixVec(self) -> 'func_adl_servicex_xaodr22.vector_float_.vector_float_'
| A method
|
| e(self) -> 'float'
| A method
|
| eta(self) -> 'float'
| A method
|
| hasNonConstStore(self) -> 'bool'
| A method
|
| hasStore(self) -> 'bool'
| A method
|
| hasValidTime(self) -> 'bool'
| A method
|
| hitPattern(self) -> 'int'
| A method
|
| index(self) -> 'int'
| A method
|
| m(self) -> 'float'
| A method
|
| numberDoF(self) -> 'float'
| A method
|
| numberOfParameters(self) -> 'int'
| A method
|
| p4(self) -> 'func_adl_servicex_xaodr22.tlorentzvector.TLorentzVector'
| A method
|
| parameterPX(self, index: 'int') -> 'float'
| A method
|
| parameterPY(self, index: 'int') -> 'float'
| A method
|
| parameterPZ(self, index: 'int') -> 'float'
| A method
|
| parameterX(self, index: 'int') -> 'float'
| A method
|
| parameterY(self, index: 'int') -> 'float'
| A method
|
| parameterZ(self, index: 'int') -> 'float'
| A method
|
| phi(self) -> 'float'
| A method
|
| phi0(self) -> 'float'
| A method
|
| pt(self) -> 'float'
| A method
|
| qOverP(self) -> 'float'
| A method
|
| radiusOfFirstHit(self) -> 'float'
| A method
|
| rapidity(self) -> 'float'
| A method
|
| theta(self) -> 'float'
| A method
|
| time(self) -> 'float'
| A method
|
| trackIndices(self) -> 'bool'
| A method
|
| usingPrivateStore(self) -> 'bool'
| A method
|
| usingStandaloneStore(self) -> 'bool'
| A method
|
| vx(self) -> 'float'
| A method
|
| vy(self) -> 'float'
| A method
|
| vz(self) -> 'float'
| A method
|
| z0(self) -> 'float'
| A method
|
| ----------------------------------------------------------------------
| Readonly properties defined here:
|
| auxdataConst
| A method
|
| isAvailable
| A method
|
| ----------------------------------------------------------------------
| Data descriptors defined here:
|
| __dict__
| dictionary for instance variables (if defined)
|
| __weakref__
| list of weak references to the object (if defined)
|
| ----------------------------------------------------------------------
| Data and other attributes defined here:
|
| covMatrixIndex = <enum 'covMatrixIndex'>
| An enumeration.
Further Information#
The
xAOD::TrackParticle_v1
C++ header file with all the inline documentation.The Tracking CP group R21 recommendation pages on the ATLAS TWiki